Quick start guide to building applications on AT Protocol
Find the source code for the example application on GitHub.
In this guide, we're going to build a simple multi-user app that publishes your current "status" as an emoji. Our application will look like this:
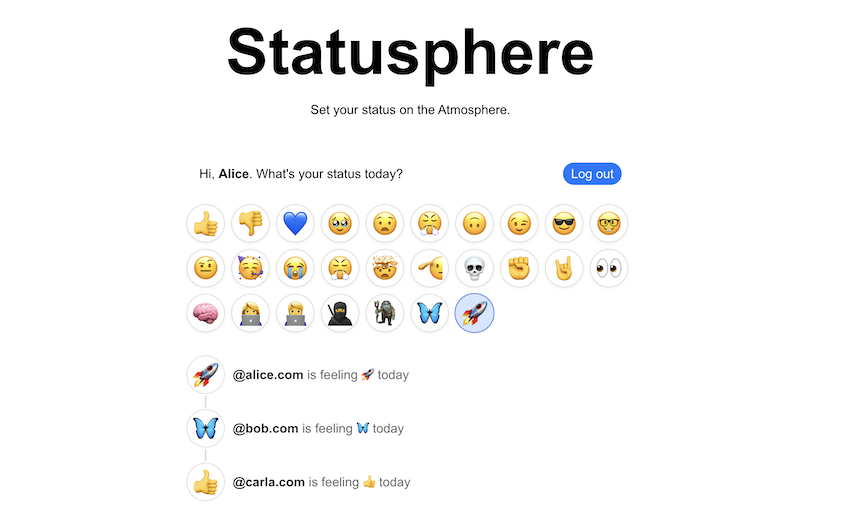
We will cover how to:
- Signin via OAuth
- Fetch information about users (profiles)
- Listen to the network firehose for new data
- Publish data on the user's account using a custom schema
We're going to keep this light so you can quickly wrap your head around ATProto. There will be links with more information about each step.
Introduction
Data in the Atmosphere is stored on users' personal repos. It's almost like each user has their own website. Our goal is to aggregate data from the users into our SQLite DB.
Think of our app like a Google. If Google's job was to say which emoji each website had under /status.json
, then it would show something like:
nytimes.com
is feeling 📰 according tohttps://nytimes.com/status.json
bsky.app
is feeling 🦋 according tohttps://bsky.app/status.json
reddit.com
is feeling 🤓 according tohttps://reddit.com/status.json
The Atmosphere works the same way, except we're going to check at://
instead of https://
. Each user has a data repo under an at://
URL. We'll crawl all the user data repos in the Atmosphere for all the "status.json" records and aggregate them into our SQLite database.
at://
is the URL scheme of the AT Protocol. Under the hood it uses common tech like HTTP and DNS, but it adds all of the features we'll be using in this tutorial.
Step 1. Starting with our ExpressJS app
Start by cloning the repo and installing packages.
git clone https://github.com/bluesky-social/statusphere-example-app.git
cd statusphere-example-app
cp .env.template .env
npm install
npm run dev
# Navigate to http://localhost:8080
Our repo is a regular Web app. We're rendering our HTML server-side like it's 1999. We also have a SQLite database that we're managing with Kysely.
Our starting stack:
With each step we'll explain how our Web app taps into the Atmosphere. Refer to the codebase for more detailed code — again, this tutorial is going to keep it light and quick to digest.
Step 2. Signing in with OAuth
When somebody logs into our app, they'll give us read & write access to their personal at://
repo. We'll use that to write the status json record.
We're going to accomplish this using OAuth (spec). Most of the OAuth flows are going to be handled for us using the @atproto/oauth-client-node library. This is the arrangement we're aiming toward:
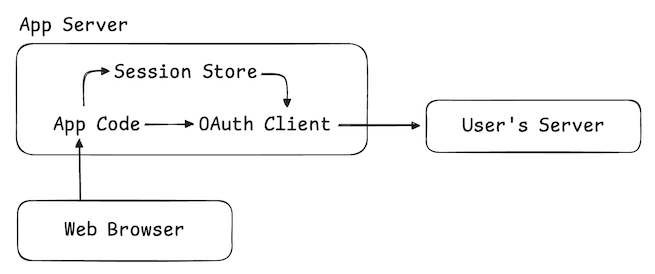
When the user logs in, the OAuth client will create a new session with their repo server and give us read/write access along with basic user info.
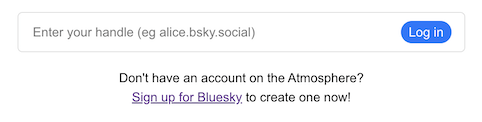
Our login page just asks the user for their "handle," which is the domain name associated with their account. For Bluesky users, these tend to look like alice.bsky.social
, but they can be any kind of domain (eg alice.com
).
<!-- src/pages/login.ts -->
<form action="/login" method="post" class="login-form">
<input
type="text"
name="handle"
placeholder="Enter your handle (eg alice.bsky.social)"
required
/>
<button type="submit">Log in</button>
</form>
When they submit the form, we tell our OAuth client to initiate the authorization flow and then redirect the user to their server to complete the process.
/** src/routes.ts **/
// Login handler
router.post(
'/login',
handler(async (req, res) => {
// Initiate the OAuth flow
const handle = req.body?.handle
const url = await oauthClient.authorize(handle, {
scope: 'atproto transition:generic',
})
return res.redirect(url.toString())
})
)
This is the same kind of SSO flow that Google or GitHub uses. The user will be asked for their password, then asked to confirm the session with your application.
When that finishes, the user will be sent back to /oauth/callback
on our Web app. The OAuth client will store the access tokens for the user's server, and then we attach their account's DID to the cookie-session.
/** src/routes.ts **/
// OAuth callback to complete session creation
router.get(
'/oauth/callback',
handler(async (req, res) => {
// Store the credentials
const { session } = await oauthClient.callback(params)
// Attach the account DID to our user via a cookie
const cookieSession = await getIronSession(req, res)
cookieSession.did = session.did
await cookieSession.save()
// Send them back to the app
return res.redirect('/')
})
)
With that, we're in business! We now have a session with the user's repo server and can use that to access their data.
Step 3. Fetching the user's profile
Why don't we learn something about our user? In Bluesky, users publish a "profile" record which looks like this:
interface ProfileRecord {
displayName?: string // a human friendly name
description?: string // a short bio
avatar?: BlobRef // small profile picture
banner?: BlobRef // banner image to put on profiles
createdAt?: string // declared time this profile data was added
// ...
}
You can examine this record directly using atproto-browser.vercel.app. For instance, this is the profile record for @bsky.app.
We're going to use the Agent associated with the user's OAuth session to fetch this record.
await agent.com.atproto.repo.getRecord({
repo: agent.assertDid, // The user
collection: 'app.bsky.actor.profile', // The collection
rkey: 'self', // The record key
})
When asking for a record, we provide three pieces of information.
- repo The DID which identifies the user,
- collection The collection name, and
- rkey The record key
We'll explain the collection name shortly. Record keys are strings with some restrictions and a couple of common patterns. The "self"
pattern is used when a collection is expected to only contain one record which describes the user.
Let's update our homepage to fetch this profile record:
/** src/routes.ts **/
// Homepage
router.get(
'/',
handler(async (req, res) => {
// If the user is signed in, get an agent which communicates with their server
const agent = await getSessionAgent(req, res, ctx)
if (!agent) {
// Serve the logged-out view
return res.type('html').send(page(home()))
}
// Fetch additional information about the logged-in user
const { data: profileRecord } = await agent.com.atproto.repo.getRecord({
repo: agent.assertDid, // our user's repo
collection: 'app.bsky.actor.profile', // the bluesky profile record type
rkey: 'self', // the record's key
})
// Serve the logged-in view
return res
.type('html')
.send(page(home({ profile: profileRecord.value || {} })))
})
)
With that data, we can give a nice personalized welcome banner for our user:

<!-- pages/home.ts -->
<div class="card">
${profile
? html`<form action="/logout" method="post" class="session-form">
<div>
Hi, <strong>${profile.displayName || 'friend'}</strong>.
What's your status today?
</div>
<div>
<button type="submit">Log out</button>
</div>
</form>`
: html`<div class="session-form">
<div><a href="/login">Log in</a> to set your status!</div>
<div>
<a href="/login" class="button">Log in</a>
</div>
</div>`}
</div>
Step 4. Reading & writing records
You can think of the user repositories as collections of JSON records:
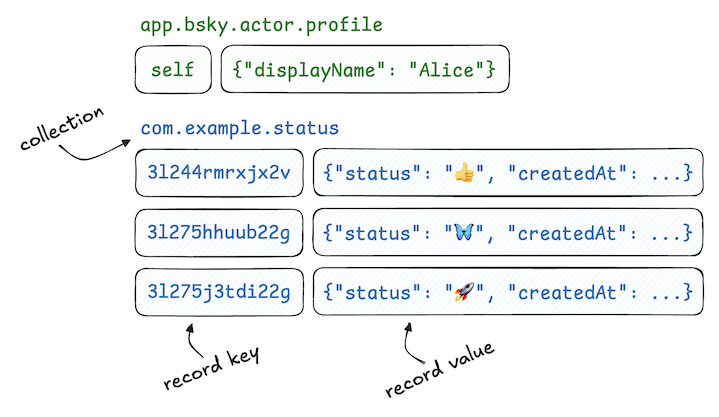
Let's look again at how we read the "profile" record:
await agent.com.atproto.repo.getRecord({
repo: agent.assertDid, // The user
collection: 'app.bsky.actor.profile', // The collection
rkey: 'self', // The record key
})
We write records using a similar API. Since our goal is to write "status" records, let's look at how that will happen:
// Generate a time-based key for our record
const rkey = TID.nextStr()
// Write the
await agent.com.atproto.repo.putRecord({
repo: agent.assertDid, // The user
collection: 'xyz.statusphere.status', // The collection
rkey, // The record key
record: { // The record value
status: "👍",
createdAt: new Date().toISOString()
}
})
Our POST /status
route is going to use this API to publish the user's status to their repo.
/** src/routes.ts **/
// "Set status" handler
router.post(
'/status',
handler(async (req, res) => {
// If the user is signed in, get an agent which communicates with their server
const agent = await getSessionAgent(req, res, ctx)
if (!agent) {
return res.status(401).type('html').send('<h1>Error: Session required</h1>')
}
// Construct their status record
const record = {
$type: 'xyz.statusphere.status',
status: req.body?.status,
createdAt: new Date().toISOString(),
}
try {
// Write the status record to the user's repository
await agent.com.atproto.putRecord({
repo: agent.assertDid,
collection: 'xyz.statusphere.status',
rkey: TID.nextStr(),
record,
})
} catch (err) {
logger.warn({ err }, 'failed to write record')
return res.status(500).type('html').send('<h1>Error: Failed to write record</h1>')
}
res.status(200).json({})
})
)
Now in our homepage we can list out the status buttons:
<!-- src/pages/home.ts -->
<form action="/status" method="post" class="status-options">
${STATUS_OPTIONS.map(status => html`
<button class="status-option" name="status" value="${status}">
${status}
</button>
`)}
</form>
And here we are!
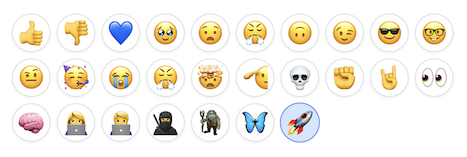
Step 5. Creating a custom "status" schema
Repo collections are typed, meaning that they have a defined schema. The app.bsky.actor.profile
type definition can be found here.
Anybody can create a new schema using the Lexicon language, which is very similar to JSON-Schema. The schemas use reverse-DNS IDs which indicate ownership. In this demo app we're going to use xyz.statusphere
which we registered specifically for this project (aka statusphere.xyz).
Why create a schema?
Schemas help other applications understand the data your app is creating. By publishing your schemas, you make it easier for other application authors to publish data in a format your app will recognize and handle.
Let's create our schema in the /lexicons
folder of our codebase. You can read more about how to define schemas here.
/** lexicons/status.json **/
{
"lexicon": 1,
"id": "xyz.statusphere.status",
"defs": {
"main": {
"type": "record",
"key": "tid",
"record": {
"type": "object",
"required": ["status", "createdAt"],
"properties": {
"status": {
"type": "string",
"minLength": 1,
"maxGraphemes": 1,
"maxLength": 32
},
"createdAt": {
"type": "string",
"format": "datetime"
}
}
}
}
}
}
Now let's run some code-generation using our schema:
./node_modules/.bin/lex gen-server ./src/lexicon ./lexicons/*
This will produce Typescript interfaces as well as runtime validation functions that we can use in our app. Here's what that generated code looks like:
/** src/lexicon/types/xyz/statusphere/status.ts **/
export interface Record {
status: string
createdAt: string
[k: string]: unknown
}
export function isRecord(v: unknown): v is Record {
return (
isObj(v) &&
hasProp(v, '$type') &&
(v.$type === 'xyz.statusphere.status#main' || v.$type === 'xyz.statusphere.status')
)
}
export function validateRecord(v: unknown): ValidationResult {
return lexicons.validate('xyz.statusphere.status#main', v)
}
Let's use that code to improve the POST /status
route:
/** src/routes.ts **/
import * as Status from '#/lexicon/types/xyz/statusphere/status'
// ...
// "Set status" handler
router.post(
'/status',
handler(async (req, res) => {
// ...
// Construct & validate their status record
const record = {
$type: 'xyz.statusphere.status',
status: req.body?.status,
createdAt: new Date().toISOString(),
}
if (!Status.validateRecord(record).success) {
return res.status(400).json({ error: 'Invalid status' })
}
// ...
})
)
Step 6. Listening to the firehose
So far, we have:
- Logged in via OAuth
- Created a custom schema
- Read & written records for the logged in user
Now we want to fetch the status records from other users.
Remember how we referred to our app as being like Google, crawling around the repos to get their records? One advantage we have in the AT Protocol is that each repo publishes an event log of their updates.
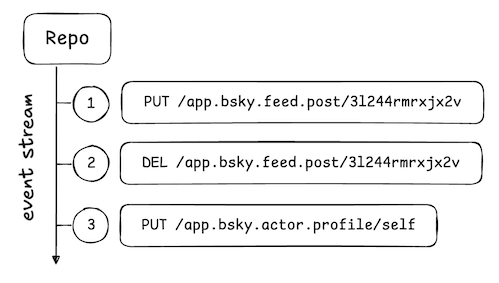
Using a Relay service we can listen to an aggregated firehose of these events across all users in the network. In our case what we're looking for are valid xyz.statusphere.status
records.
/** src/ingester.ts **/
import { Firehose } from '@atproto/sync'
import * as Status from '#/lexicon/types/xyz/statusphere/status'
// ...
new Firehose({
filterCollections: ['xyz.statusphere.status'],
handleEvent: async (evt) => {
// Watch for write events
if (evt.event === 'create' || evt.event === 'update') {
const record = evt.record
// If the write is a valid status update
if (
evt.collection === 'xyz.statusphere.status' &&
Status.isRecord(record) &&
Status.validateRecord(record).success
) {
// Store the status
// TODO
}
}
},
})
Let's create a SQLite table to store these statuses:
/** src/db.ts **/
// Create our statuses table
await db.schema
.createTable('status')
.addColumn('uri', 'varchar', (col) => col.primaryKey())
.addColumn('authorDid', 'varchar', (col) => col.notNull())
.addColumn('status', 'varchar', (col) => col.notNull())
.addColumn('createdAt', 'varchar', (col) => col.notNull())
.addColumn('indexedAt', 'varchar', (col) => col.notNull())
.execute()
Now we can write these statuses into our database as they arrive from the firehose:
/** src/ingester.ts **/
// If the write is a valid status update
if (
evt.collection === 'xyz.statusphere.status' &&
Status.isRecord(record) &&
Status.validateRecord(record).success
) {
// Store the status in our SQLite
await db
.insertInto('status')
.values({
uri: evt.uri.toString(),
authorDid: evt.author,
status: record.status,
createdAt: record.createdAt,
indexedAt: new Date().toISOString(),
})
.onConflict((oc) =>
oc.column('uri').doUpdateSet({
status: record.status,
indexedAt: new Date().toISOString(),
})
)
.execute()
}
You can almost think of information flowing in a loop:
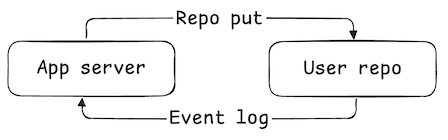
Applications write to the repo. The write events are then emitted on the firehose where they're caught by the apps and ingested into their databases.
Why sync from the event log like this? Because there are other apps in the network that will write the records we're interested in. By subscribing to the event log, we ensure that we catch all the data we're interested in — including data published by other apps!
Step 7. Listing the latest statuses
Now that we have statuses populating our SQLite, we can produce a timeline of status updates by users. We also use a DID-to-handle resolver so we can show a nice username with the statuses:
/** src/routes.ts **/
// Homepage
router.get(
'/',
handler(async (req, res) => {
// ...
// Fetch data stored in our SQLite
const statuses = await db
.selectFrom('status')
.selectAll()
.orderBy('indexedAt', 'desc')
.limit(10)
.execute()
// Map user DIDs to their domain-name handles
const didHandleMap = await resolver.resolveDidsToHandles(
statuses.map((s) => s.authorDid)
)
// ...
})
)
Our HTML can now list these status records:
<!-- src/pages/home.ts -->
${statuses.map((status, i) => {
const handle = didHandleMap[status.authorDid] || status.authorDid
return html`
<div class="status-line">
<div>
<div class="status">${status.status}</div>
</div>
<div class="desc">
<a class="author" href="https://bsky.app/profile/${handle}">@${handle}</a>
was feeling ${status.status} on ${status.indexedAt}.
</div>
</div>
`
})}
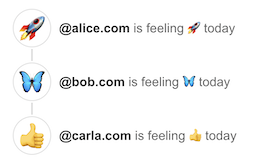
Step 8. Optimistic updates
As a final optimization, let's introduce "optimistic updates."
Remember the information flow loop with the repo write and the event log?
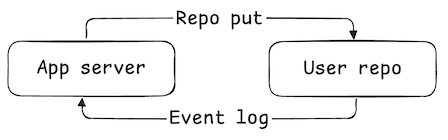
Since we're updating our users' repos locally, we can short-circuit that flow to our own database:
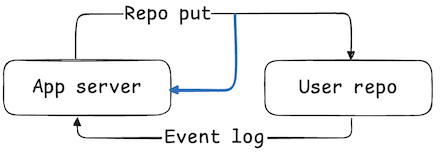
This is an important optimization to make, because it ensures that the user sees their own changes while using your app. When the event eventually arrives from the firehose, we just discard it since we already have it saved locally.
To do this, we just update POST /status
to include an additional write to our SQLite DB:
/** src/routes.ts **/
// "Set status" handler
router.post(
'/status',
handler(async (req, res) => {
// ...
let uri
try {
// Write the status record to the user's repository
const res = await agent.com.atproto.repo.putRecord({
repo: agent.assertDid,
collection: 'xyz.statusphere.status',
rkey: TID.nextStr(),
record,
})
uri = res.uri
} catch (err) {
logger.warn({ err }, 'failed to write record')
return res.status(500).json({ error: 'Failed to write record' })
}
try {
// Optimistically update our SQLite <-- HERE!
await db
.insertInto('status')
.values({
uri,
authorDid: agent.assertDid,
status: record.status,
createdAt: record.createdAt,
indexedAt: new Date().toISOString(),
})
.execute()
} catch (err) {
logger.warn(
{ err },
'failed to update computed view; ignoring as it should be caught by the firehose'
)
}
res.status(200).json({})
})
)
You'll notice this code looks almost exactly like what we're doing in ingester.ts
.
Thinking in AT Proto
In this tutorial we've covered the key steps to building an atproto app. Data is published in its canonical form on users' at://
repos and then aggregated into apps' databases to produce views of the network.
When building your app, think in these four key steps:
- Design the Lexicon schemas for the records you'll publish into the Atmosphere.
- Create a database for aggregating the records into useful views.
- Build your application to write the records on your users' repos.
- Listen to the firehose to aggregate data across the network.
Remember this flow of information throughout:
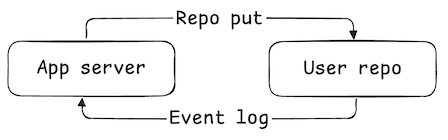
This is how every app in the Atmosphere works, including the Bluesky social app.
Next steps
If you want to practice what you've learned, here are some additional challenges you could try:
- Sync the profile records of all users so that you can show their display names instead of their handles.
- Count the number of each status used and display the total counts.
- Fetch the authed user's
app.bsky.graph.follow
follows and show statuses from them. - Create a different kind of schema, like a way to post links to websites and rate them 1 through 4 stars.